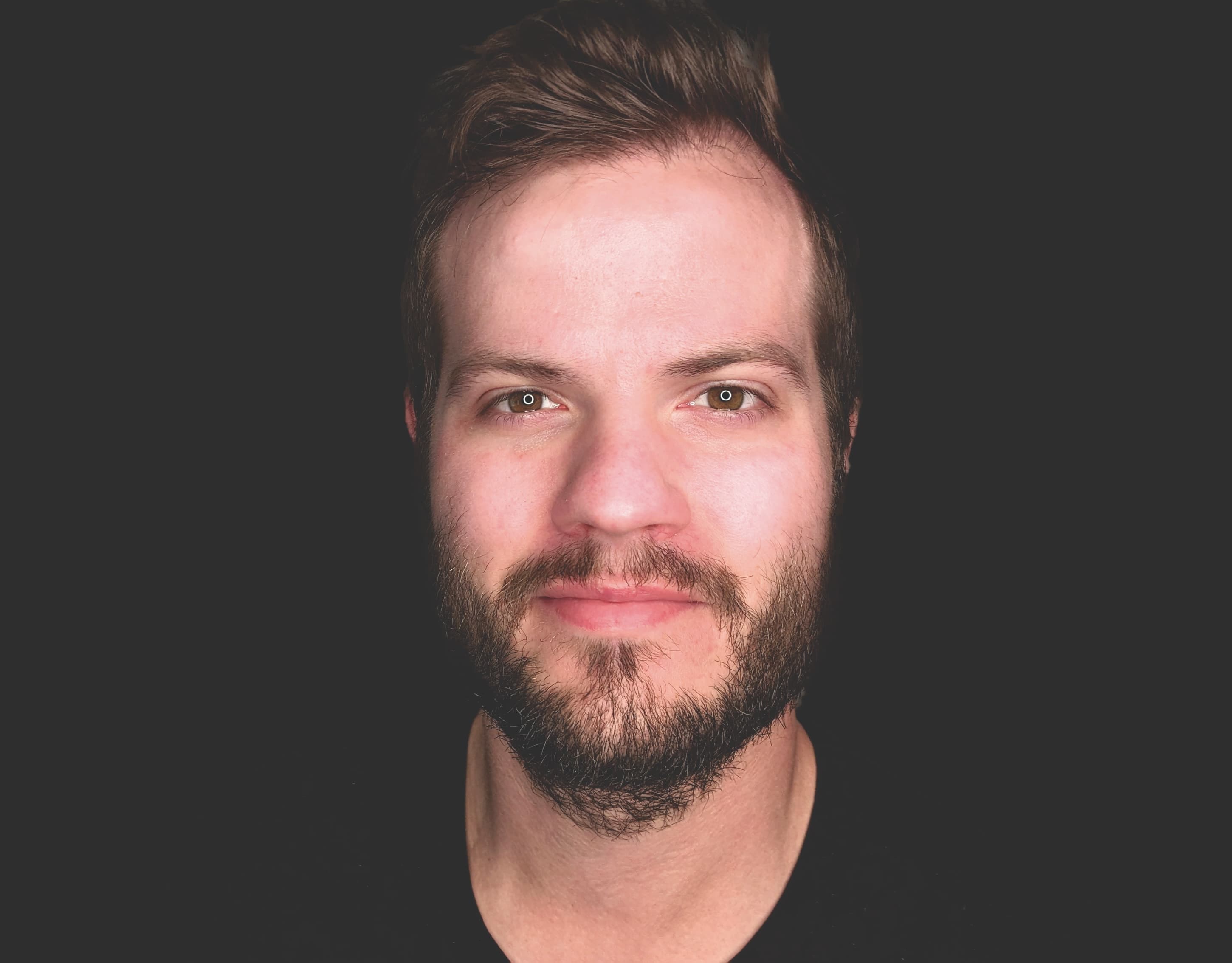
February 5, 2024
•Last updated February 7, 2024
Create a Responsive Tailwind Navbar with Dropdowns
Creating a responsive navigation bar is crucial for any website, and Tailwind CSS offers a streamlined, utility-first approach to achieve this.
This guide will walk you through constructing a simple Tailwind navbar component with a dropdown menu. We’ll also ensure the navigation is adaptable across all devices.
Why Tailwind CSS for Responsive Navbars?
Tailwind CSS simplifies building responsive navbars by providing utility classes that can be applied directly in your HTML, eliminating the need for extensive custom CSS. Whether you aim to implement a Tailwind navbar with dropdown functionalities or just a navigation component, Tailwind has you covered.
Table of Contents
- Step 1: Add the HTML for a Simple Tailwind Navbar
- Step 2: Responsive Design with Tailwind CSS
- Step 3: Toggling the Dropdown Menu
- Step 4: Toggling the Tailwind Navbar mobile menu
- Step 5: Customizing and Testing Your Tailwind Navbar
Prerequisites
- Basic understanding of
HTML
andCSS
- Tailwind CSS integrated into your project
Step 1: Add the HTML for a Simple Tailwind Navbar
The foundation of our Tailwind navbar component begins with its HTML structure. Here's a straightforward layout to start with:
<nav class="bg-gray-800 text-white">
<div class="container mx-auto px-4 md:flex items-center gap-6">
<!-- Logo -->
<div class="flex items-center justify-between md:w-auto w-full">
<a href="#" class="flex items-center py-5 px-2 text-white flex-1">
<span class="font-bold">Webcrunch</span>
</a>
<!-- Mobile Menu Button -->
<div class="md:hidden flex items-center">
<button class="mobile-menu-button">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24">
<title>bars-3-bottom-left</title>
<g fill="none">
<path d="M3.75 6.75h16.5M3.75 12h16.5m-16.5 5.25H12" stroke="currentColor" stroke-width="1.5" stroke-linecap="round" stroke-linejoin="round"></path>
</g>
</svg>
</button>
</div>
</div>
<!-- Primary Navigation -->
<div class="hidden md:flex md:flex-row flex-col items-center justify-start md:space-x-1 navigation-menu pb-3 md:pb-0 navigation-menu">
<a href="#" class="py-2 px-3 block">Home</a>
<a href="#" class="py-2 px-3 block">About</a>
<!-- Dropdown Menu -->
<div class="relative">
<button class="dropdown-toggle py-2 px-3 hover:bg-gray-700 flex items-center gap-2 rounded">
<span class="pointer-events-none">Services</span>
<svg class="w-3 h-3 pointer-events-none" xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24">
<title>chevron-down</title>
<g fill="none">
<path d="M19.5 8.25l-7.5 7.5-7.5-7.5" stroke="currentColor" stroke-width="1.5" stroke-linecap="round" stroke-linejoin="round"></path>
</g>
</svg>
</button>
<div class="dropdown-menu absolute hidden bg-gray-700 text-white rounded-b-lg pb-2 w-48">
<a href="#" class="block px-6 py-2 hover:bg-gray-800">Web Design</a>
<a href="#" class="block px-6 py-2 hover:bg-gray-800">Development</a>
<a href="#" class="block px-6 py-2 hover:bg-gray-800">SEO</a>
</div>
</div>
<a href="#" class="py-2 px-3 block">Contact</a>
</div>
</div>
</nav>
Step 2: Responsive Design with Tailwind CSS
To ensure our Tailwind navbar is responsive, we utilize Tailwind's built-in classes like md:flex
for medium screens and hidden
to toggle visibility. These utilities allow the navbar to adapt seamlessly across different devices.
Step 3: Toggling the Dropdown Menu
For the dropdown menu within our Tailwind navbar component, we can add a bit of JavaScript to handle the interaction:
document.addEventListener("DOMContentLoaded", () => {
// Select all dropdown toggle buttons
const dropdownToggles = document.querySelectorAll(".dropdown-toggle")
dropdownToggles.forEach((toggle) => {
toggle.addEventListener("click", () => {
// Find the next sibling element which is the dropdown menu
const dropdownMenu = toggle.nextElementSibling
// Toggle the 'hidden' class to show or hide the dropdown menu
if (dropdownMenu.classList.contains("hidden")) {
// Hide any open dropdown menus before showing the new one
document.querySelectorAll(".dropdown-menu").forEach((menu) => {
menu.classList.add("hidden")
})
dropdownMenu.classList.remove("hidden")
} else {
dropdownMenu.classList.add("hidden")
}
})
})
// Optional: Clicking outside of an open dropdown menu closes it
window.addEventListener("click", (event) => {
if (!event.target.matches(".dropdown-toggle")) {
document.querySelectorAll(".dropdown-menu").forEach((menu) => {
if (!menu.contains(event.target)) {
menu.classList.add("hidden")
}
})
}
})
})
Step 4: Toggling the Tailwind Navbar mobile menu
// Mobile menu toggle
const mobileMenuButton = document.querySelector(".mobile-menu-button")
const mobileMenu = document.querySelector(".navigation-menu")
mobileMenuButton.addEventListener("click", () => {
mobileMenu.classList.toggle("hidden")
})
On mobile screens, we hide the menu from view and display a hamburger menu icon. This icon is tapable on smaller screens. The javascript targets both the mobile menu and th
Step 5: Customizing and Testing Your Tailwind Navbar
Tailwind CSS makes building a navigation component a breeze and offers endless customization options to ensure your navbar aligns perfectly with your brand's aesthetics. Let's dive deeper into how you can personalize your navbar with features like sticky navigation and dark mode support and why testing across different devices is crucial for user experience.
Customizing the Tailwind Navbar
- Color Schemes: Tailwind provides various color utilities. You can change your navbar's background, text, and hover states to match your brand colors. For instance, use
bg-blue-500
for a blue background ortext-white
for white text. Don't forget to apply hover effects with classes likehover:bg-blue-700
for interactive elements. - Spacing and Layout: Tailwind's spacing utilities allow you to adjust padding and margin for the perfect layout. Use classes like
px-4
(padding on the x-axis) ormy-2
(margin on the y-axis) to space elements within your navbar. - Typography: Customize the text size, weight, and color using Tailwind's typography classes. For example,
text-lg
for larger text orfont-bold
for bold text can make your navbar items stand out.
Implementing a Tailwind Sticky Nav
To make your navbar sticky, meaning it stays at the top of the viewport as the user scrolls, add the sticky
and top-0
classes to your <nav>
element. This ensures that your navigation is always accessible, regardless of where the user is on the page:
<nav class="sticky top-0 bg-gray-800 text-white z-50">
The z-50
class ensures your navbar stays above other content. Adjust the z-index
as needed based on your page's elements.
Adding Dark Mode Support
Tailwind CSS v2.0 and above supports dark mode out of the box, making it easy for users who prefer a darker interface to adapt your navbar design. Use the dark:
prefix to specify different styles for dark mode:
<nav class="bg-gray-800 text-white dark:bg-black dark:text-gray-200">
In this example, the navbar will have a black background and lighter text in dark mode. Refer to Tailwind's documentation for proper dark mode configuration. You can define dark mode based on a global body class name or support device-enabled dark mode by default.
Testing Across Devices
- Responsiveness: Use browser dev tools to simulate various screen sizes and ensure your navbar looks good on all devices. Pay special attention to breakpoints where your layout changes, such as when the mobile menu button appears.
- Interactivity: Test interactive elements like dropdown menus and links to ensure they work as expected on touch devices and with mouse and keyboard.
- Performance: Ensure your navbar performs well, especially when implementing features like sticky positioning or dynamic dark mode switching, which can impact rendering.
- Accessibility: Test for accessibility, ensuring your navbar is navigable using keyboard shortcuts and screen readers. This includes proper ARIA labels for interactive elements.
Conclusion
With Tailwind CSS, creating a simple, responsive navbar component with a dropdown menu becomes a pretty straightforward process. By leveraging Tailwind's utility classes, you can make a nav bar that's both stylish and functional across all screen sizes.
Continue reading
Categories
Collection
Part of the Tailwind CSS collection