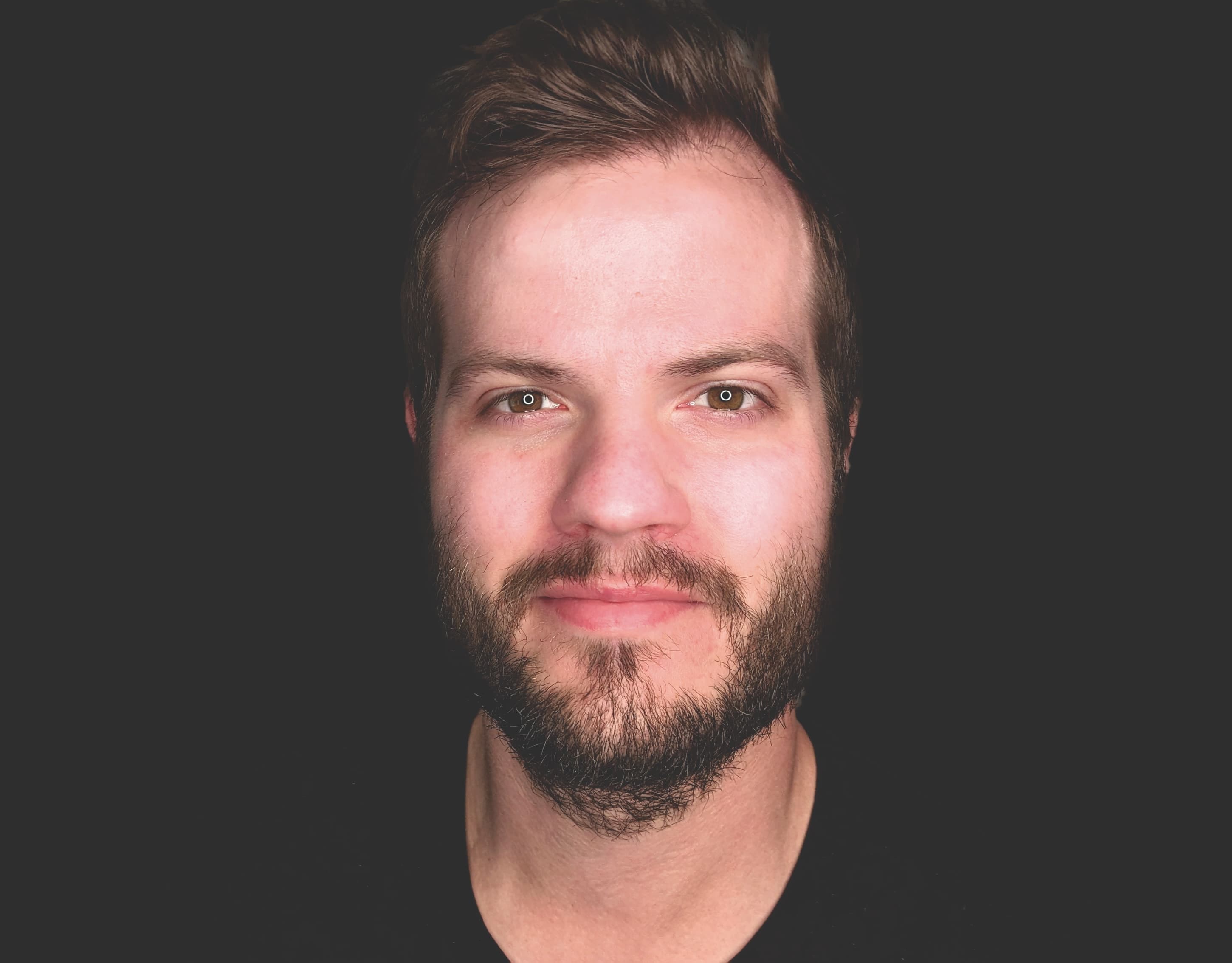
August 16, 2018
•Last updated November 5, 2023
Let's Build: With JavaScript - Broadcast Bar with Cookies
I'm back at it with the Let's Build: With JavaScript series. In this video learn how to build a broadcast bar with cookies using vanilla JavaScript and some elbow grease.
Slowly but surely I'll be contributing to this series more as I find realistic examples worthy of teaching. I like to learn by solving problems. Building things rather than just reading books, following long-form blog post tutorials and the occasional video tutorial makes a larger impact. In many cases, those who author these "help" documents don't actually teach anything other than the language itself. Rather than go down that path, I strive to create real examples. I do this to better understand the problem and solution. I also get to teach you at the same time. Win-win!
This particular example utilizes some browser technologies to create a cookie for any user who navigates to your page and closes a broadcast banner. If they did indeed click the "X" icon, a cookie gets stored for 7 days in their browser.
This solution isn't foolproof as a user can just clear their cookies to see the broadcast bar again, but it does allow you to leverage storing something without needing a user to be signed in to some sort of session.
Give this a shot on your own and see what you come up with.
HTML
<div class="broadcast">
<div class="container"> Help improve Affinicasts by taking a short survey. <a href="https://andyleverenz1.typeform.com/to/vdwDSK" target="_blank">Add your response</a>
</div>
<div class="hide-broadcast">
<a href="#" class="hide-broadcast-target">
<svg width="20" height="20" viewBox="0 0 20 20"><path d="M10.707 10.5l5.646-5.646a.5.5 0 0 0-.707-.707L10 9.793 4.354 4.147a.5.5 0 0 0-.707.707L9.293 10.5l-5.646 5.646a.5.5 0 0 0 .708.707l5.646-5.646 5.646 5.646a.498.498 0 0 0 .708 0 .5.5 0 0 0 0-.707L10.709 10.5z"></path></svg>
</a>
</div>
</div>
<div class="moar-content container">
<p>
Lorem ipsum dolor sit amet consectetur adipisicing elit. Maiores dolorem ea similique ullam, ipsam explicabo facere facilis, quibusdam cupiditate suscipit eaque commodi alias sint? Minus consequuntur deleniti quas quia nesciunt.
</p>
<p>Lorem ipsum dolor sit, amet consectetur adipisicing elit. Consequatur nemo unde dignissimos dolore vitae provident optio molestiae excepturi quae fugiat nisi libero nulla, nesciunt repellat odio quia ullam? Sequi, voluptate.</p>
</div>
SCSS
@import url('https://fonts.googleapis.com/css?family=Muli');
$brand-secondary: #F3B823;
$text-color: #444;
* {
margin: 0;
padding: 0;
}
.container {
max-width: 1170px;
margin: 0 auto;
}
.broadcast {
padding: .6rem 0;
color: white;
display: flex;
text-align: center;
font-family: 'Muli', sans-serif;
}
.broadcast {
background: #444;
position: relative;
font-weight: bold;
a {
color: $brand-secondary;
text-decoration: none;
}
.hide-broadcast {
position: absolute;
right: 20px;
top: 3px;
svg {
fill: white;
width: 20px;
height: 20px;
position: relative;
top: 2px;
}
.hide-broadcast-target {
width: 24px;
height: 24px;
display: inline-block;
border-radius: 50%;
padding: 4px;
transition: all ease .3s;
}
&:hover {
.hide-broadcast-target {
background: rgba(white, .9);
svg { fill: $text-color; }
}
}
}
}
.moar-content {
padding: 3rem 0;
font-size: 16px;
font-family: "Muli", sans-serif;
p {
margin-bottom: 1.6rem;
}
}
JavaScript
The main focus of this tutorial is this code.
var broadcast = document.querySelector('.broadcast');
var closeBroadcast = document.querySelector('.hide-broadcast-target');
var cookie = readCookie('hide_broadcast_bar');
if (cookie == "true") {
broadcast.style.display = "none";
}
closeBroadcast.addEventListener('click', function(e) {
if(cookie != "true") {
broadcast.style.display = "none";
createCookie('hide_broadcast_bar', "true", 7);
}
e.preventDefault();
});
function createCookie(name, value, days) {
if(days) {
var date = new Date();
date.setTime(date.getTime() + (days * 24 * 60 * 60 * 1000));
var expires = "; expires=" + date.toGMTString();
} else var expires = "";
document.cookie = name + "=" + value + expires + "; path=/"
}
function readCookie(name) {
var nameEQ = name + "=";
var ca = document.cookie.split(';');
for (var i = 0; i < ca.length; i++) {
var c = ca[i];
while (c.charAt(0) == ' ') c = c.substring(1, c.length);
if (c.indexOf(nameEQ) == 0) return c.substring(nameEQ.length, c.length);
}
return null;
}
function eraseCookie(name) {
createCookie(name, "", -1);
}
Check out the series so far
Categories
Collection
Part of the Let's Build: With JavaScript collection