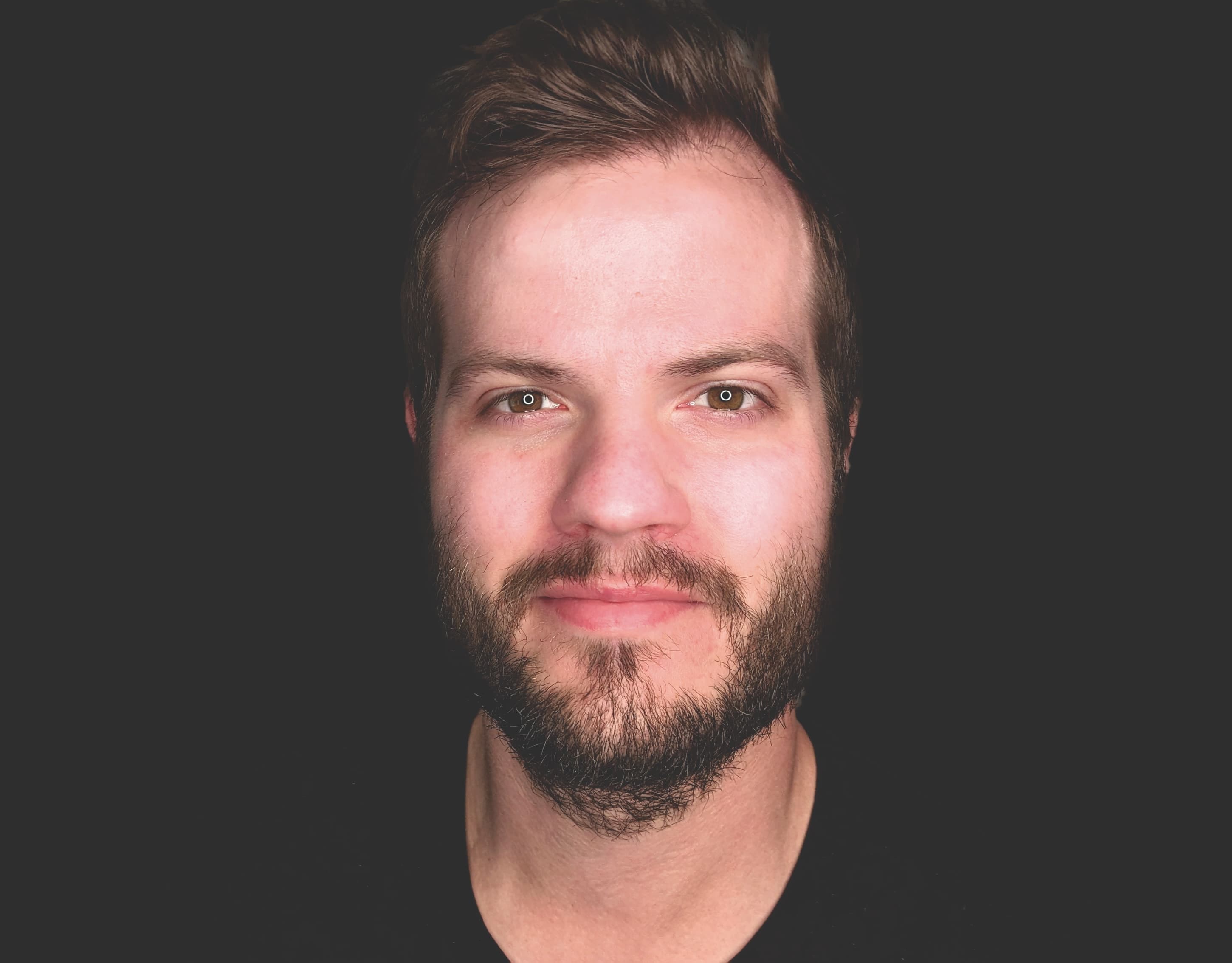
May 31, 2023
•Last updated November 5, 2023
Address Already in Use: Resolving the "Bind Error" in Ruby on Rails
If you've used Rails for a length of time you've likely gotten an error of this type: "Address already in use - bind(2) (Errno::EADDRINUSE)"
Running multiple instances of a Ruby on Rails application on the same port can sometimes lead to the dreaded "Address already in use bind error." This error occurs when two or more applications try to bind to the same port, causing a conflict. In this article, we will explore the causes behind this error and provide effective solutions to resolve it, allowing you to run multiple instances of your Ruby on Rails app successfully.
Understanding the "Address already in use bind error":
The "Address already in use bind error" occurs when another process or application already uses a port.
In Ruby on Rails, each application instance must bind to a unique port to avoid conflicts. The error is triggered when two instances attempt to bind to the same port simultaneously.
The TL;DR:
lsof -wni tcp:3000
That might return this:
COMMAND PID USER FD TYPE DEVICE SIZE/OFF NODE NAME
ruby 3366 dummy 8u IPv4 16901 0t0 TCP 127.0.0.1:3000 (LISTEN)
To kill the process, pass the PID to this line:
kill -9 3366
Solutions to the "Address already in use bind error":
1. Specify different ports:
The most straightforward solution is to ensure that each instance of your Ruby on Rails application binds to a different port. By default, Rails uses port 3000. To specify a different port, you can start your application with the following command:
rails s -p <port_number>
Replace <port_number>
with the desired port, such as 3001
, 3002
, and so on. This allows you to run multiple application instances on different ports without conflicts.
2. Check for running processes:
Sometimes, the error may occur even when you believe no other instances of your application are running. In such cases, checking for any lingering processes that might be using the port is essential. You can use the following command to find the processes running on a specific port (e.g., port 3000):
lsof -wni tcp:3000
The command will provide a list of processes using that port. Identify the unwanted processes and terminate them using the appropriate system command (e.g., kill <pid>
on Unix-based systems – If you have a Mac, this applies to you too).
3. Delayed startup:
Another approach to avoiding the bind error is introducing a delay in each instance's startup process. By staggering the start times, you can ensure that each instance can bind to its respective port before the next instance attempts to bind. This can be achieved by adding a sleep command before starting each instance, as shown in the following example:
# Start first instance
sleep 5
rails s -p 3000
# Start second instance
sleep 5
rails s -p 3001
Adjust the sleep time according to your requirements.
4. Use environment variables:
If you have multiple instances of your application running on the same machine frequently, you can consider using environment variables to set the port dynamically.
By doing so, each instance can automatically select an available port, reducing the likelihood of conflicts. To achieve this, you can modify your Rails application's config/boot.rb
file. Here's an example snippet that sets the port based on the APP_PORT
environment variable:
require "rails"
require "rails/commands/server"
module Rails
class Server
def default_options
super.merge!(Port: (ENV["APP_PORT"] || 3000).to_i)
end
end
end
With this mod, you can start your application by setting the APP_PORT
environment variable:
APP_PORT=3001 rails s
Each instance can bind to a unique port based on the provided environment variable.
Bonus: zsh alias power user command
You can create a Zsh alias to handle the "Address already in use bind error". Here's an example of how you can define the alias:
Open your Zsh configuration file. You can use the following command to open it in a text editor:
nano ~/.zshrc
Add the following alias at the end of the file:
alias start-rails='function _start_rails() { sleep 5 && rails s -p $1; }; _start_rails'
This alias defines a function _start_rails
that waits 5 seconds using sleep
and then starts the Rails server with the specified port. The start-rails
alias invokes this function.
Save the changes and exit the text editor.
Reload the Zsh configuration to apply the changes:
source ~/.zshrc
Now, you can use the start-rails
alias to start your Ruby on Rails application with the desired port. For example, if you want to start an instance on port 3000, you can use the following command:
start-rails 3000
Similarly, you can start another instance with a different port:
start-rails 3001
The alias will automatically introduce a delay before starting each instance, reducing the chances of encountering the "Address already in use bind error."
Note: Ensure the rails
command is available in your system's PATH for the alias to work correctly.
Categories
Collection
Part of the Ruby on Rails collection